UICollectionView在目前的iOS开发中,使用非常广泛,它可以完成许多UITableView完成不了的复杂的布局,在使用上,两者也有着许多的相似之处,主要体现在布局的样式上,UITableView主要有两种布局:plain和group,而UICollectionView则可以根据我们的需要自定义各种各样复杂的布局,这也是下面我们就UICollectionView的使用进行一下详细的解析。
基本使用方法
与UITableView相似,在UICollectionView时,也要遵循数据源(UICollectionViewDataSource)与代理协议方法(UICollectionViewDelegate),也要注册cell,与UITableView不同的是,我们还需要为UICollectionView创建一个布局参数,也就是UICollectionViewFlowLayout,这也是UICollectionView的精髓所在,正是通过它,我们才实现了UICollectionView各式各样的布局,这我们在后面会一一讲述,有兴趣的同学也可以看一下苹果官方文档。
系统为我们提供了两个UICollectionView的布局类:UICollectionViewLayout和UICollectionViewFlowLayout,UICollectionViewLayout类是一个抽象类,我们在自定义布局的时候可以继承此类,并在此基础上设置布局信息,UICollectionViewFlowLayout继承于UICollectionViewLayout,是系统为我们写好的布局类,该类为我们提供了一个简单的布局样式,假如我们只需要一个特别简单的网格布局或者流水布局,可以直接使用它。
创建UICollectionView的基本代码如下:
初始化
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17
| UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init];
UICollectionView* collectionView = [[UICollectionView alloc]initWithFrame:CGRectMake(0, 64, kScreenWidth, kScreenHeight-64) collectionViewLayout:layout]; collectionView.delegate = self; collectionView.dataSource = self; collectionView.backgroundColor = [UIColor cyanColor];
[collectionView registerClass:[MyCollectionViewCell class] forCellWithReuseIdentifier:@"MyCollectionViewCell"];
[collectionView registerClass:[UICollectionReusableView class] forSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:@"MyCollectionViewHeaderView"];
[collectionView registerClass:[UICollectionReusableView class] forSupplementaryViewOfKind:UICollectionElementKindSectionFooter withReuseIdentifier:@"MyCollectionViewFooterView"];
[self.view addSubview:collectionView];
|
实现协议方法
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76
| #pragma mark - UICollectionViewDelegate,UICollectionViewDataSource
- (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView{ return 1; }
- (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section{ return _imagesArray.count; }
- (__kindof UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath{ static NSString *cellIdentifier = @"MyCollectionViewCell"; MyCollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:cellIdentifier forIndexPath:indexPath]; cell.backgroundColor = [UIColor cyanColor]; cell.imageStr = _imagesArray[indexPath.item]; return cell; }
- (UICollectionReusableView *)collectionView:(UICollectionView *)collectionView viewForSupplementaryElementOfKind:(NSString *)kind atIndexPath:(NSIndexPath *)indexPath{ if (kind == UICollectionElementKindSectionHeader){ UICollectionReusableView *headerView = [collectionView dequeueReusableSupplementaryViewOfKind:kind withReuseIdentifier:@"MyCollectionViewHeaderView" forIndexPath:indexPath]; headerView.backgroundColor = [UIColor yellowColor]; UILabel *titleLabel = [[UILabel alloc]initWithFrame:headerView.bounds]; titleLabel.text = [NSString stringWithFormat:@"第%ld个分区的区头",indexPath.section]; [headerView addSubview:titleLabel]; return headerView; }else if(kind == UICollectionElementKindSectionFooter){ UICollectionReusableView *footerView = [collectionView dequeueReusableSupplementaryViewOfKind:kind withReuseIdentifier:@"MyCollectionViewFooterView" forIndexPath:indexPath]; footerView.backgroundColor = [UIColor blueColor]; UILabel *titleLabel = [[UILabel alloc]initWithFrame:footerView.bounds]; titleLabel.text = [NSString stringWithFormat:@"第%ld个分区的区尾",indexPath.section]; [footerView addSubview:titleLabel]; return footerView; } return nil; }
- (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath{ NSLog(@"点击了第%ld分item",(long)indexPath.item); }
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout sizeForItemAtIndexPath:(NSIndexPath *)indexPath{ CGFloat itemW = (kScreenWidth-(kLineNum+1)*kLineSpacing)/kLineNum-0.001; return CGSizeMake(itemW, itemW); }
- (UIEdgeInsets)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout insetForSectionAtIndex:(NSInteger)section{ return UIEdgeInsetsMake(kLineSpacing, kLineSpacing, kLineSpacing, kLineSpacing); }
- (CGFloat)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout minimumLineSpacingForSectionAtIndex:(NSInteger)section{ return kLineSpacing; }
- (CGFloat)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout minimumInteritemSpacingForSectionAtIndex:(NSInteger)section{ return kLineSpacing; }
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout referenceSizeForHeaderInSection:(NSInteger)section{ return CGSizeMake(kScreenWidth, 65); }
- (CGSize)collectionView:(UICollectionView *)collectionView layout:(UICollectionViewLayout*)collectionViewLayout referenceSizeForFooterInSection:(NSInteger)section{ return CGSizeMake(kScreenWidth, 65); }
|
最终的效果图如下:
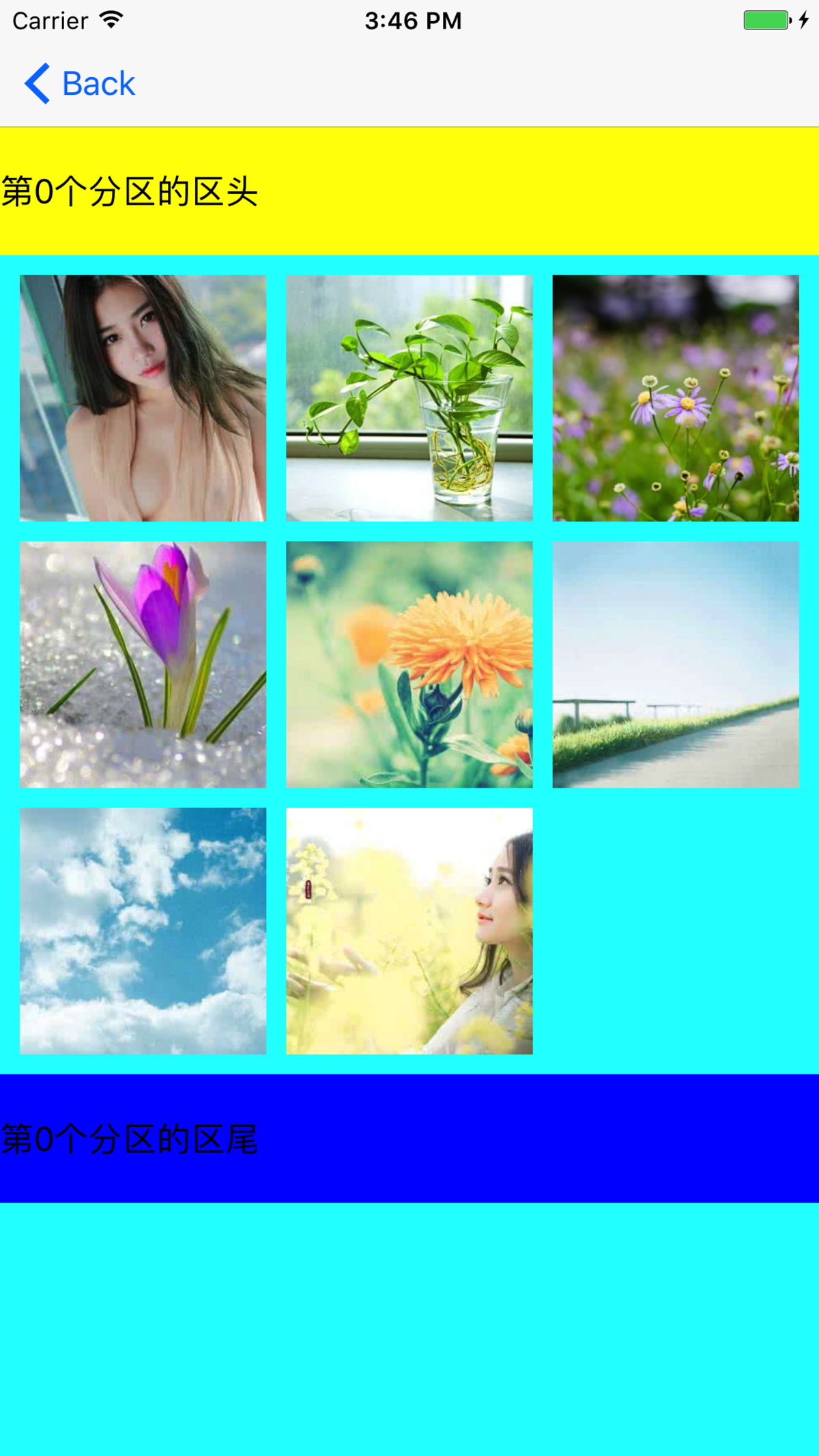
上面我们提到了,UICollectionViewFlowLayout是系统为我们提供的一个简单的流水布局,那么我们在直接使用它做简单的布局时,可以直接设置它的一个属性,来达到我们布局的目的,这样就可以省略掉一些UICollectionView协议方法的实现。
我们来看一下UICollectionViewFlowLayout都有哪些属性可以设置:
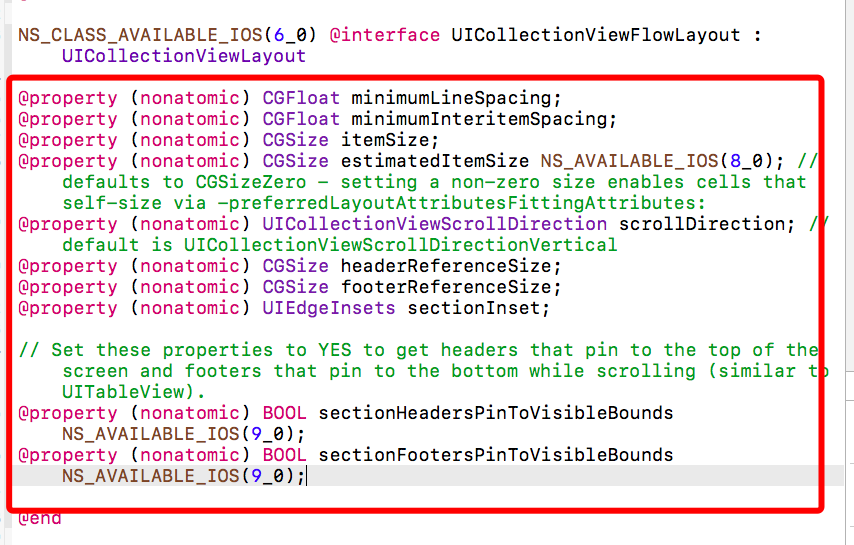
和上面我们实现的UICollectionView中的一些协议方法的名字非常相似,根据名字应该可以一一对应。
那么,使用UICollectionViewFlowLayout的属性设置布局的效果如果呢,我们来看:
代码实现
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38
|
UICollectionViewFlowLayout *layout = [[UICollectionViewFlowLayout alloc] init];
layout.minimumInteritemSpacing = kLineSpacing; layout.minimumLineSpacing = kLineSpacing;
CGFloat itemW = (kScreenWidth-(kLineNum+1)*kLineSpacing)/kLineNum-0.001; layout.itemSize = CGSizeMake(itemW, itemW);
layout.sectionInset = UIEdgeInsetsMake(kLineSpacing, kLineSpacing,kLineSpacing, kLineSpacing);
layout.headerReferenceSize = CGSizeMake(kScreenWidth, 65); layout.footerReferenceSize = CGSizeMake(kScreenWidth, 65);
layout.sectionFootersPinToVisibleBounds = YES;
UICollectionView* collectionView = [[UICollectionView alloc]initWithFrame:CGRectMake(0, 64, kScreenWidth, kScreenHeight-64) collectionViewLayout:layout]; collectionView.delegate = self; collectionView.dataSource = self; collectionView.backgroundColor = [UIColor cyanColor]; [collectionView registerClass:[MyCollectionViewCell class] forCellWithReuseIdentifier:@"MyCollectionViewCell"]; [collectionView registerClass:[UICollectionReusableView class] forSupplementaryViewOfKind:UICollectionElementKindSectionHeader withReuseIdentifier:@"MyCollectionViewHeaderView"]; [collectionView registerClass:[UICollectionReusableView class] forSupplementaryViewOfKind:UICollectionElementKindSectionFooter withReuseIdentifier:@"MyCollectionViewFooterView"]; [self.view addSubview:collectionView];
|
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37
| #pragma mark - UICollectionViewDelegate,UICollectionViewDataSource - (NSInteger)numberOfSectionsInCollectionView:(UICollectionView *)collectionView{ return 1; } - (NSInteger)collectionView:(UICollectionView *)collectionView numberOfItemsInSection:(NSInteger)section{ return _imagesArray.count; } - (__kindof UICollectionViewCell *)collectionView:(UICollectionView *)collectionView cellForItemAtIndexPath:(NSIndexPath *)indexPath{ static NSString *cellIdentifier = @"MyCollectionViewCell"; MyCollectionViewCell *cell = [collectionView dequeueReusableCellWithReuseIdentifier:cellIdentifier forIndexPath:indexPath]; cell.backgroundColor = [UIColor cyanColor]; cell.imageStr = _imagesArray[indexPath.item]; return cell; } - (UICollectionReusableView *)collectionView:(UICollectionView *)collectionView viewForSupplementaryElementOfKind:(NSString *)kind atIndexPath:(NSIndexPath *)indexPath{ if (kind == UICollectionElementKindSectionHeader){ UICollectionReusableView *headerView = [collectionView dequeueReusableSupplementaryViewOfKind:kind withReuseIdentifier:@"MyCollectionViewHeaderView" forIndexPath:indexPath]; headerView.backgroundColor = [UIColor yellowColor]; UILabel *titleLabel = [[UILabel alloc]initWithFrame:headerView.bounds]; titleLabel.text = [NSString stringWithFormat:@"第%ld个分区的区头",indexPath.section]; [headerView addSubview:titleLabel]; return headerView; }else if(kind == UICollectionElementKindSectionFooter){ UICollectionReusableView *footerView = [collectionView dequeueReusableSupplementaryViewOfKind:kind withReuseIdentifier:@"MyCollectionViewFooterView" forIndexPath:indexPath]; footerView.backgroundColor = [UIColor blueColor]; UILabel *titleLabel = [[UILabel alloc]initWithFrame:footerView.bounds]; titleLabel.text = [NSString stringWithFormat:@"第%ld个分区的区尾",indexPath.section]; [footerView addSubview:titleLabel]; return footerView; } return nil; } - (void)collectionView:(UICollectionView *)collectionView didSelectItemAtIndexPath:(NSIndexPath *)indexPath{ NSLog(@"点击了第%ld分item",(long)indexPath.item); }
|
效果图:

事实证明,两种方法在实现的效果上并没有任何区别。
总结
关于UICollectionView的基本使用大概就是这些内容,但是这些只适用于一些简单的网格或流水布局,如果遇到比较复杂的布局,比如瀑布流等,就需要自定义UICollectionViewLayout来布局了。在下一篇文章中,我们会详细介绍如何使用自定义UICollectionViewLayout来编写复杂布局。